Welcome to the 2nd of Prisync API Tutorial Series aiming to clarify the API foundations.
In the first blog post, I speculated about:
- which problems Prisync (and the API V2.0) solves on behalf of you,
- creating the API key and token,
- adding your first product via API V2.0,
- adding your URL and a bunch of competitor URLs,
- and batch importing via the API.
Purpose – Prisync API Tutorial
In this post, I’ll go through gathering the data using Prisync API V2.0. Yet, you can re-visit the first post of Prisync API Tutorial Series and API documentation.
First things first, I’ll assume that you followed the first post and you’ve already added a couple of products to your Prisync account. No worries if you don’t have any products. Just go back and complete the first tutorial or you can add products on your dashboard. Besides, we’ll need the make_request method that we used in the first post so that let me recap it below:
It’s a tiny method which calls the API endpoints, adding the authentication headers.
Listing the Products You Have
Prisync API V2.0 has /list/product endpoint, which basically lists all products on your account.
All you need to do is making a request to the /list/product endpoint:
The response is below:
VoilĂ ! I got all the products (since I only have 4 of them) in just one request. results is a JSON array, returning the list of products up to 100 items. When you have more than 100 products, the result is paginated by 100. Therefore in every response, the API returns the next page’s URL so that you can iterate within a for a loop.
Each item in the results list represents a single product. So each product contains:
- ID
- Name
- Category (both name and category ID)
- Brand (both name and brand ID)
- Product Code (SKU, EAN or any other internal product code)
Each product on your Prisync account has several URLs including your own URL and competitor URLs. Another step we reached, we now need to retrieve those URLs.
This is easy to do. What you need to do is calling the get a particular product endpoint. Let’s call it for the “iPhone X, GSM Unlocked 5.8 – 64 GB – Space Gray“. I need the product id of it and it lays down in the /list/product request response:
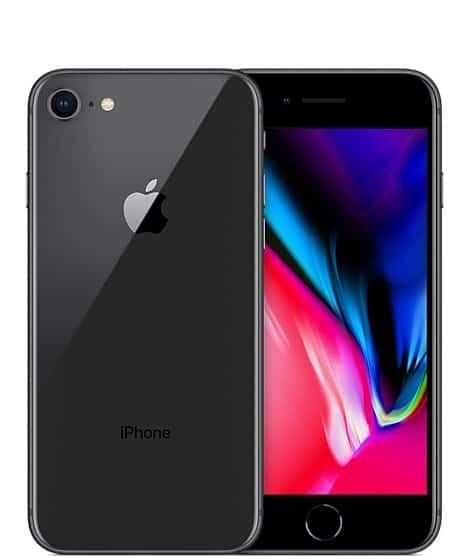
“id”: “2055805” “name”: “iPhone X, GSM Unlocked 5.8 – 64 GB – Space Gray”,
Getting a particular product
Seems like we are almost there. Let’s put the ID into the URL and call make_request method:
https://gist.github.com/prisync/dfa24921f00cdb1eed478b3889fa2652
Here is the response:
This endpoint returns more details about the product, such as product_cost, external_ref, smart_price and URLs. Consequently we are interested in URLs, so we’ll dive into others in next posts.
Since we want to focus on urls field let’s dig it up. It contains an array of integers, each pointing to a URL belonging to this product. In this product, there are 4 URLs whose IDs are 8273503, 8273504, 8273505 and 8273506.
Due to we have IDs of the URLs, next step is calling get a specific URL
endpoint.
Getting a Particular URL
It is just a simple for loop iterating over all URL IDs and getting URL details:
…and here is the output of the piece of code:
… (there are 3 other URL data, so I just removed them for the sake of simplicity.)
In the URL details, there is several fields and most of them self explanatory. For the details of each field, you can look up in the API docs.
Since we have all the competitor prices, it is easy to write a piece of code to find the items which got more than 10% discounts today:
Finally, the output is:
Discount alert at https://www.coolblue.nl/product/793610/apple-iphone-x-64gb-zilver.html
And finally we know that coolblue.nl discounted the price more than 10% today.
To sum up all the code of this post:
There are numerous things to do if you have access to the competitor prices, which Prisync can proudly present. Just to mention a few of them:
- First of all you can monitor your competitors actively and generate custom alerts depending on your pricing strategy.
- in addition to that, set your prices when the market is stale so that you can create volatility, which directly affects your profit margins.
- Another feature you can develop is ignoring discounts if the stock is not available on the competitor.
- in addition, you can implement other ideas that you and your team come up with!
Thanks for following up to this point. This is the second post of our API article series. See you in next posts of Prisync API Tutorial Series.
prisync
Leave a Reply